Sometimes you want to open your app from another app. Or maybe the user taps on a URL on their device and you want it to load your app.
It’s pretty easy…
We’ll use 2 apps for this.
- OpenMe – We want to open this app via a URL
- OpenOtherApp – This app will open the OpenMe app
OpenMe App
In the OpenMe app, go to the Info tab. Under the URL Types, click the + button.
Put the app’s bundle identifier (e.g., com.example.OpenMe) in the Identifier field.
In the URL Schemes field, put in the scheme. For most links we use in browsers, the scheme is HTTP/S. In this case it will be openme.
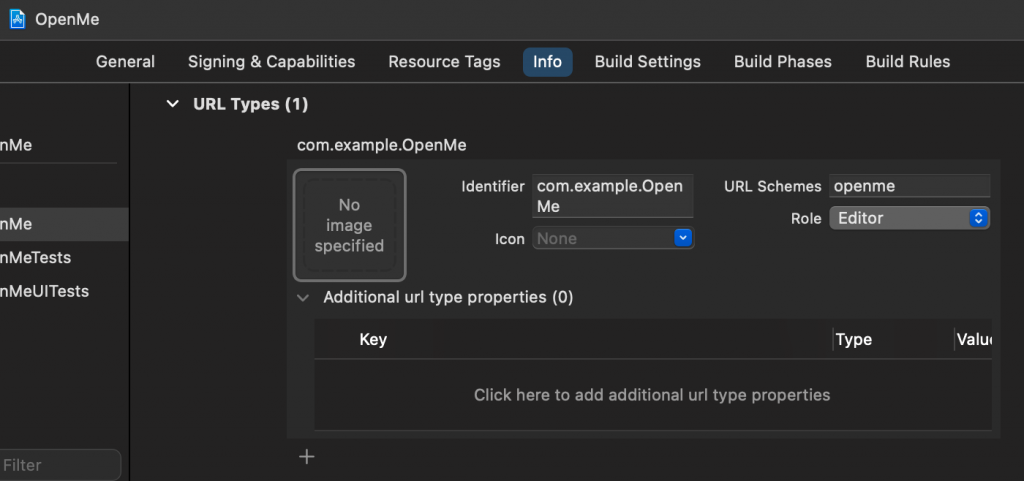
That’s if for the OpenMe app. Run it in the simulator so that it’s set to be opened.
In the OpenOtherApp code, you want to open a URL with that scheme.
OpenOtherApp App
We just want to add some code to open the URL. I have it tied to an action on a button:
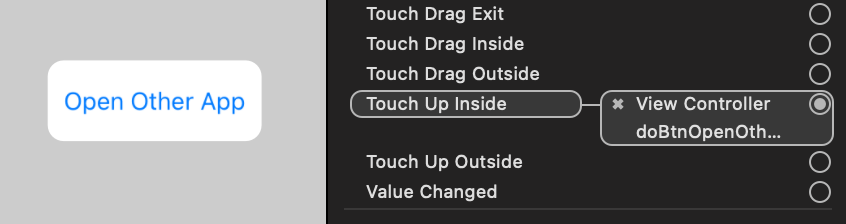
@IBAction func doBtnOpenOtherApp(_ sender: Any) {
guard let url = URL.init(string: "openme://")
else { return }
UIApplication.shared.open(url,
options: [:])
{ (success) in
print ("opening...")
}
}
First we create the URL in a guard. Finally, we open the URL which launches the other app.
Run this app in the same simulator and tap the button.
A bit more…
In some cases, you’ll want to verify that the URL can be launched before trying to launch it. In that case, you want to add this line before the call to open the URL:
guard UIApplication.shared.canOpenURL(url) else { return }
If you run OpenOtherApp at this point, the line above will return false. That’s because you haven’t told the app this URL scheme is supported.
To add this as a supported scheme to OpenOtherApp, add LSApplicationQueriesSchemes to your Info.plist:

Or as text in your Info.plist opened as source code:
<key>LSApplicationQueriesSchemes</key>
<array>
<string>openme</string>
</array>
Now the canOpenURL will return true and running the app will open the OpenMe app.
Passing Parameters in the URL
If you use parameters in your URL, you can access them in a SceneDelegate function like this:
func scene(_ scene: UIScene,
openURLContexts URLContexts: Set<UIOpenURLContext>) {
print (URLContexts.first?.url.absoluteString ?? "NO URL")
}
I hope that helps!